How to integrate with Stitch using GraphQL
With this article, we aim to provide useful code examples to demystify GraphQL and give you the tools you need to succeed with your Stitch GraphQL integration.
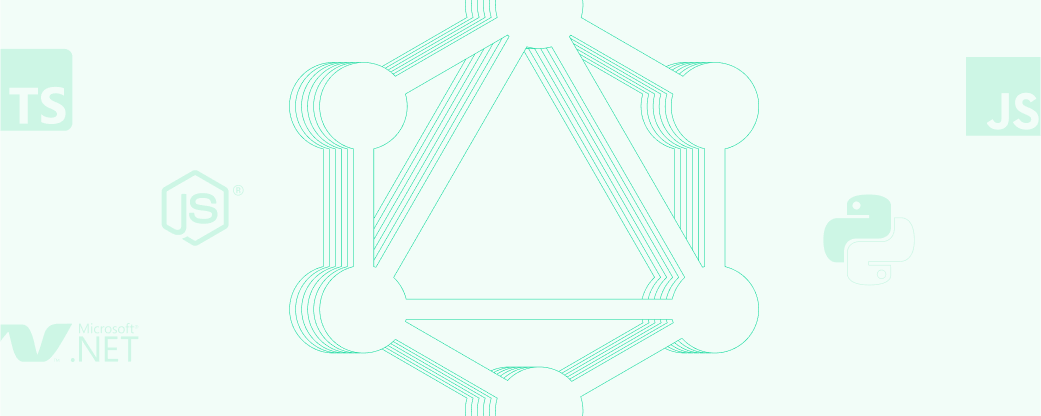
As infrastructure and connection become increasingly critical to enabling the growth of tech businesses, we’re seeing more API solutions than ever before. Since the early 2000s, one of the key standards in API design has been REST. Since then, web applications have become increasingly more powerful and reactive. Modern apps can run on mobile or in a browser seamlessly. To support this new style of rich interaction, GraphQL was developed at Facebook and released in 2015.
GraphQL’s unique selling points are:
- Everything is typed You get type-safety and a clearly defined schema as part of the spec.
- Query only for what you need It’s possible to query only the precise data you need to achieve your goals. The result is lower latencies, less data usage, and faster queries.
- Everything is connected Interrelated data can be queried directly using that relationship. This means that if you query a bank account by account number, for example, you can also query the transactions associated with that bank account at the same time.
Stitch chose GraphQL as the paradigm for our API because it makes queries faster and simpler, and it makes integrating with a frontend much easier because you only ever get what you ask for.
Why did Stitch choose GraphQL and what is it?
Using an API can be frustrating if you have no idea what data to expect from it or what shape it’ll be in when you get it. GraphQL side-steps these issues by requiring that the schema and the relationships between data are defined beforehand.
Having a defined structure for all the types of data you can access makes it easy to code against because the types can be relied on.
GraphQL is a structured query language. What that means is you aren’t just calling an endpoint as you would with a REST API; you’re actually asking the server to return specific data in a specific structure. You define what data you want back and you can for the most part decide what shape it will be returned in. At Stitch we also use our own API as part of our system - it’s not just for external use!
To illustrate the difference, here is an example of a comparison between REST and GraphQL when querying bank account information and associated transactions for a specific user. In our example, we wanted the descriptions and amounts of all transactions associated with all of the user’s ABSA bank accounts.
Rest
# First we get the list of accounts
GET /users/1/absa/accounts
[
{
"name": "ABSA Savings Account",
"balance": 50.49,
"currency": "ZAR",
"status": "active",
"accountNumber": "123456789",
"accountType": "savings"
},
{
"name": "ABSA Check Account",
"balance": 150.72,
"currency": "ZAR",
"status": "active",
"accountNumber": "987654321",
"accountType": "check"
}
]
# We have to get the transactions for both accounts separately
GET /users/1/absa/transactions/123456789
[]
GET /users/1/absa/transactions/987654321
[
{
"description": "Salary Payment",
"amount": 15000,
"currency": "ZAR",
"timestamp": "2022-02-25T15:33:55"
}
]}
In this case, we would have to make three separate queries to get all the data we wanted. We then end up with a lot of extra data back by default.
Obviously, REST has ways of solving these issues, but many of them are workarounds that shoehorn these features into a paradigm that doesn’t support them. REST is great for many applications, but for web frontends or complicated queries where only specific data is important, GraphQL really shines!
Now let’s do the same query but using GraphQL.
GraphQL
# POST api.stitch.money/graphql
query userTransactions {
user {
bankAccounts {
name
transactions {
edges {
node {
amount
description
}
}
}
}
}
}
The GraphQL query above is a real query you can use in the Stitch IDE right now. Copy it into the left pane, select “Test User Credential” from the Credential dropdown and click the play button to see it in action. To see more queries you can run in our IDE, check out the “Common Queries” page in the Stitch Docs.
Result:
{
"data": {
"user": {
"bankAccounts": [
{
"name": "ABSA Savings Account",
"transactions": {
"edges": []
}
},
{
"name": "ABSA Check Account",
"transactions": {
"edges": [
{
"node": {
"amount": {
"quantity": "15000.00",
"currency": "ZAR"
},
"description": "Salary Payment"
}
}
]
}
}
]
}
}
}
As you can see we got back exactly the data we asked for and nothing extra. GraphQL also has the benefit of returning the data in the same shape as the query you used to ask for it! This makes it easy to write code to parse the results.
However, GraphQL isn’t just for retrieving data using Queries; you can also update, insert and delete data using Mutations.
It’s simple to use once you understand what’s going on and very easy to reason about when writing code that uses it. The benefits of computing and development speed that we get from the flexibility of the querying system and the assurances we get from the typed schema make GraphQL an invaluable part of Stitch’s tech stack.
How can I use GraphQL to integrate with Stitch?
GraphiQL
The first step on your integration journey is going to be the GraphiQL interface available at https://stitch.money/ide.
This is the easiest way to get started to determine the structure of the queries and mutations you need to build your app. On your first visit it’ll look like this:
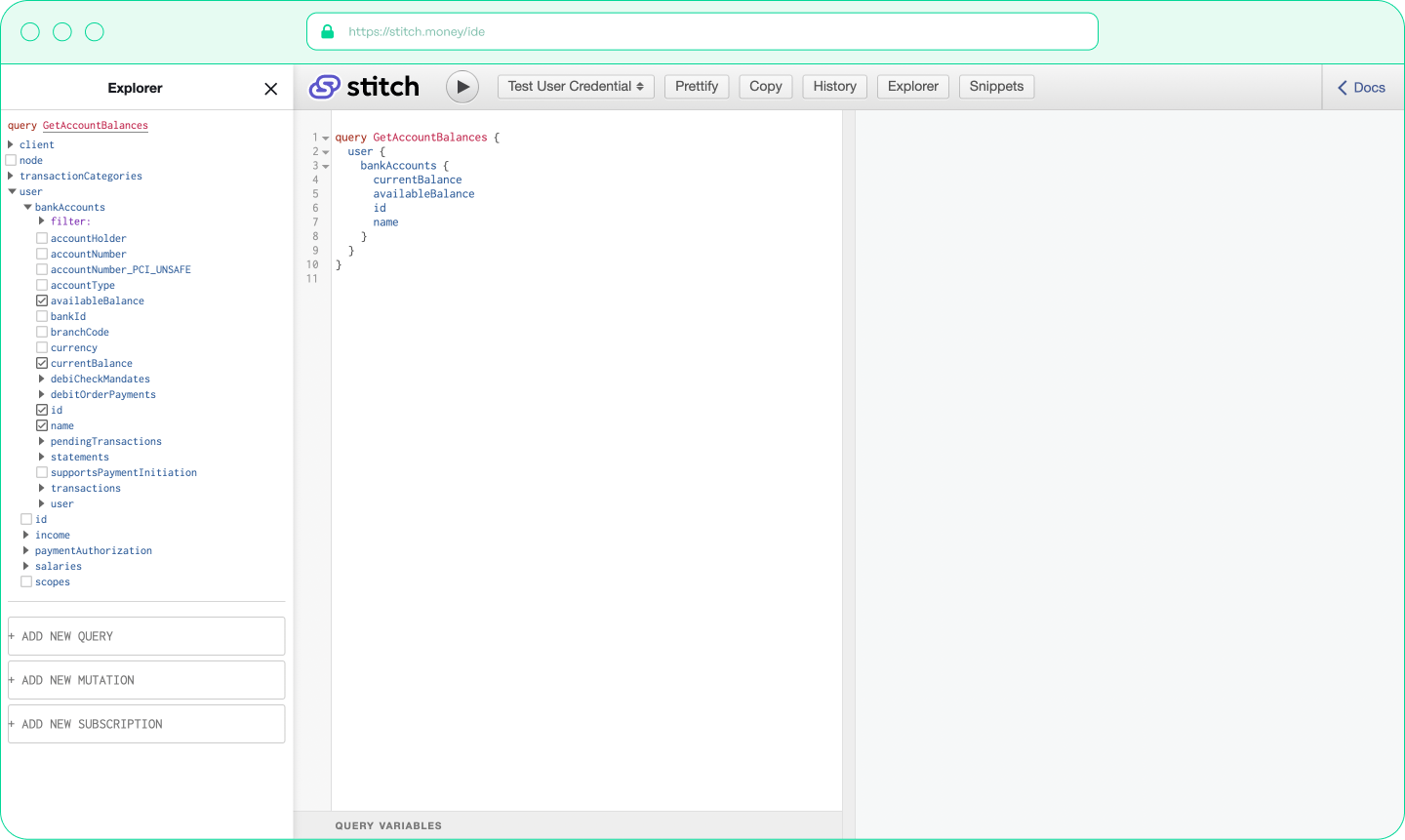
You will do all your query and mutation prototyping in the middle pane. Just write out your query, or design it using the interactive builder under the heading “Explorer”, select your credentials from the dropdown and click the play button to run it. The results will show up in the right pane.
To find out more about the queries, mutations and types in the Stitch API schema, click the button in the top right labelled “Docs”. This will open the “Documentation Explorer” tab.
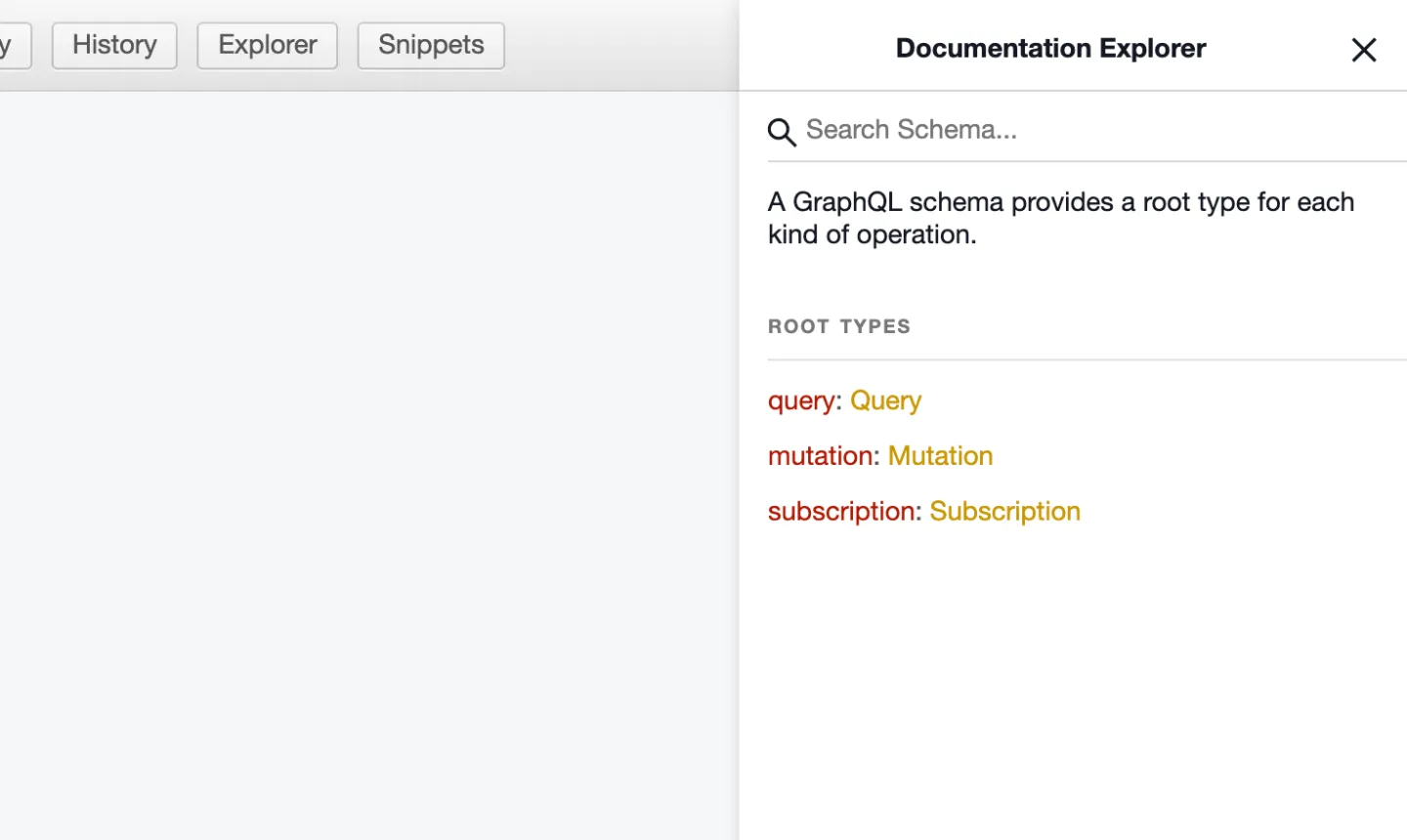
You can click on the types, queries or mutations to see their definitions and any related types.
For more information on the IDE, you can check out the related “Using the IDE” documentation.
Using GraphiQL is a good way to get familiar with the Stitch API, its features and capabilities and how GraphQL works. Our “Test Client Credential” and “Test User Credential” will allow you to try things out without any additional setup.
Once you’re ready to move on, the next step is taking the queries you’ve created using GraphiQL and moving them into code. The next section will walk through how to make the three most common queries we see from our clients in the top four programming languages our clients use.
Code integration
To follow along in this section you either need to use the test credentials provided by Stitch on signup or your production credentials.
To get test credentials, use our self-serve signup.
The following queries are the ones our clients use the most. For each query we’ll provide the query and any setup code required to run it against the Stitch API and get the results.
There will be an example for each in NodeJS (JavaScript & TypeScript), .Net, and Python.
If you don’t already have a GraphQL client library for your programming language of choice you can refer to the official listing of libraries by programming language. In our examples, we’ll use one of the options from the list for each language.
Get balances
Query (Interactive Example)
query GetAccountBalances {
user {
bankAccounts {
currentBalance
availableBalance
id
name
}
}
}
Get Transactions
Query (Interactive Example)
query TransactionsByBankAccount($accountId: ID!, $first: UInt, $after: Cursor) {
node(id: $accountId) {
... on BankAccount {
transactions(first: $first, after: $after) {
pageInfo {
hasNextPage
endCursor
}
edges {
node {
id
amount
reference
description
date
runningBalance
}
}
}
}
}
}
Create a Payment Request
Query (Interactive Example)
mutation CreatePaymentRequest(
$amount: MoneyInput!,
$payerReference: String!,
$beneficiaryReference: String!,
$externalReference: String,
$beneficiaryName: String!,
$beneficiaryBankId: BankBeneficiaryBankId!,
$beneficiaryAccountNumber: String!) {
clientPaymentInitiationRequestCreate(input: {
amount: $amount,
payerReference: $payerReference,
beneficiaryReference: $beneficiaryReference,
externalReference: $externalReference,
beneficiary: {
bankAccount: {
name: $beneficiaryName,
bankId: $beneficiaryBankId,
accountNumber: $beneficiaryAccountNumber
}
}
}) {
paymentInitiationRequest {
id
url
}
}
}
Conclusion
GraphQL allows developers to be precise about the data they need with each query. It provides type-safety, a defined known schema with types, and a simple-to-use graphical interface. Building with GraphQL is a simple, intuitive process if you have the right tools – like GraphiQL for experimentation or any of the libraries we covered in the code integration section.
We encourage anyone interested in Stitch and our product offering to check out the Stitch IDE. It’s a vehicle for exploration and queries that you write there can be transferred directly into code.
Go forth and build! We at Stitch look forward to seeing what you create.